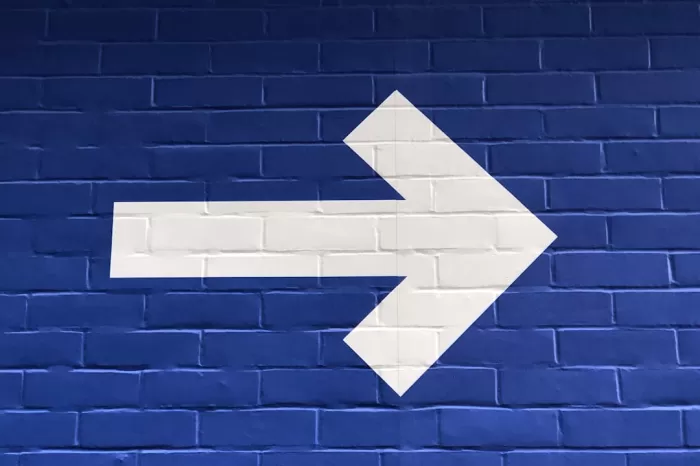
Choosing the Right Framework
React
React is a popular JavaScript library for building user interfaces. It allows you to create reusable UI components and efficiently update the UI when data changes. For example, you can create a Button component and reuse it across your application.
Angular
Angular is a comprehensive framework developed by Google. It provides tools for building large-scale applications with features like routing, forms, and HTTP client. For instance, you can use Angular's HttpClient to make API calls and fetch data from a server.
Vue
Vue is a progressive JavaScript framework that is known for its simplicity and ease of integration. It allows you to build interactive interfaces with components and handle state management effectively. You can create a TodoList component in Vue to manage a list of tasks.
- Consider the size and complexity of your project when choosing a framework.
- Evaluate the learning curve and community support for each framework.
- Experiment with a few frameworks to see which one aligns best with your development style.
Implementing Infinite Scrolling Functionality
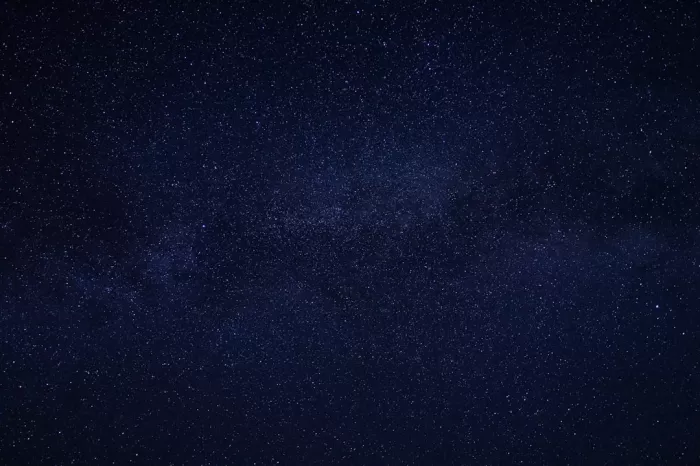
Welcome to our tutorial on how to implement infinite scrolling functionality on a website!
Step 1: Set up your HTML structure
First, you need to have a container where your content will be loaded dynamically. This can be a <div>
element with a specific ID, for example:
<div id="content"></div>
Step 2: Load initial content
Next, you will need to load some initial content into the container. This can be achieved using JavaScript or any back-end technology:
<script> // Example JavaScript to load initial content const content = document.getElementById('content'); content.innerHTML = 'Initial content here...'; </script>
Step 3: Implement the infinite scrolling logic
Now comes the exciting part! You will need to detect when the user has scrolled to the bottom of the page and then load more content dynamically. Here's a basic example using JavaScript:
<script> window.addEventListener('scroll', function() { if (window.innerHeight + window.scrollY >= document.body.offsetHeight) { // Load more content here } }); </script>
Step 4: Load more content
Finally, when the user reaches the bottom of the page, you can load more content into the container. This can be achieved by making an AJAX call to fetch more data and appending it to the existing content:
<script> // Example AJAX call to fetch more content const moreContent = 'More dynamic content here...'; content.innerHTML += moreContent; </script>
And that's it! You have successfully implemented infinite scrolling on your website. Happy coding!